React is a javascript library for building UI’s. It’s a different approach, usually people use templates. With react, you work with javascript objects. I think is a good idea, but, it’s better to do similar implementation using grooscript.
I wrote this guide where I created a DSL on top of Node.js using traits. Now I’m going to use AST’s and groovy beans. Use the groovy features in the browser, is the main reason to use grooscript.
So let’s create reactive objects as react.js does, in a TO-DO sample application:
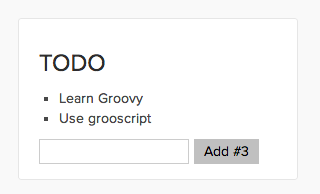
In javascript, from react.js page
var TodoList = React.createClass({displayName: 'TodoList',
render: function() {
var createItem = function(itemText) {
return React.DOM.li(null, itemText);
};
return React.DOM.ul(null, this.props.items.map(createItem));
}
});
var TodoApp = React.createClass({displayName: 'TodoApp',
getInitialState: function() {
return {items: [], text: ''};
},
onChange: function(e) {
this.setState({text: e.target.value});
},
handleSubmit: function(e) {
e.preventDefault();
var nextItems = this.state.items.concat([this.state.text]);
var nextText = '';
this.setState({items: nextItems, text: nextText});
},
render: function() {
return (
React.DOM.div(null,
React.DOM.h3(null, "TODO"),
TodoList({items: this.state.items}),
React.DOM.form({onSubmit: this.handleSubmit},
React.DOM.input({onChange: this.onChange, value: this.state.text}),
React.DOM.button(null, 'Add #' + (this.state.items.length + 1))
)
)
);
}
});
React.renderComponent(TodoApp(null), mountNode);
In groovy, my implementation
package react
@Component (1)
class TodoApp {
List<String> todos (2)
String actualTodo (3)
void init() { (4)
todos = []
actualTodo = ''
}
void actualTodoChange(actualTodoValue) { (5)
setActualTodo(actualTodoValue)
}
void addTodosSubmit() { (6)
if (actualTodo) {
todos << actualTodo
setActualTodo('')
}
}
void render() { (7)
form(id: 'addTodos') {
h3 'TODO'
ul {
todos.each {
li it
}
li {
input(type: 'text', id: 'actualTodo', value: actualTodo)
button {
yield "Add #${todos.size() + 1}"
}
}
}
}
}
}
1 | AST that do all react job. |
2 | List of all TODO’s added. |
3 | New TODO that is being typed in the text input. |
4 | Initialization of the component. |
5 | React on any change in the input text. |
6 | On 'Add TODO' click. |
7 | Html dsl that renders the component. |
For the execution in javascript, I have used this groovy script (react.groovy):
import org.grooscript.jquery.GQueryImpl (1)
import react.TodoApp
def renderComponent = { component, selector ->
def gQuery = new GQueryImpl()
gQuery.onReady {
component.start(selector) (4)
}
}
renderComponent(new TodoApp(), '#todos') (5)
1 | Little helper class that comes with grooscript to do some jQuery stuff. |
2 | Call init function in the component. |
3 | Set the selector where draw the component. |
4 | Start the component. |
5 | Call the function to render the component on load the page. |
Advantages
-
You can create your own framework / libraries, that perfect fit with your application.
-
You write groovy code and test it with spock or your favorite java / groovy testing framework.
-
You don’t have to do functional tests, you can test all with unit tests and mock javascript / jquery.
-
You write the render code as a DSL, using groovy code, very powerful.
-
You can use traits, AST’s, a little of inheritance, DSL’s, a bit of meta-programming,… and a lot of groovy magic in your application.
-
You don’t have to use any pre-processor as react.js does, just converting the code to javascript applies the AST’s.
Implementation
The code is implemented as part of the grooscript-demos project on github, and you
can see in action in grooscript homepage. Just with grooscript I convert TodoApp.groovy
and react.groovy
files
to javascript and add them with grooscript.js
, grooscript-tools.js
and jquery.js
to html:
<html>
<head>
<title>Initial static web page</title>
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/grooscript.min.js"></script>
<script type="text/javascript" src="js/grooscript-tools.js"></script>
<script type="text/javascript" src="js/react.js"></script>
</head>
<body>
<div id="todos"></div>
</body>
You can take a look at the source code on Github
Conclusion
Implement things in groovy is a pleasure, and now, with grooscript, you can create nice pieces of code that will run smooth in your browser. This is just a suggestion, loads of new ideas can be created in the browser or node.js using groovy and grooscript.
Please, if you have comments, suggestions, problems,… don’t hesitate to contact me at grooscript@gmail.com, or open an issue or feature in Github. More guides come in the future, also can find more documentation in grooscript site.